A rxjs buffer operator collects the values emitted from a source Observable as an array and emits them whenever the inner observable emits a notification. This operator is available under the package rxjs/operators
.
Marble diagram of the buffer operator
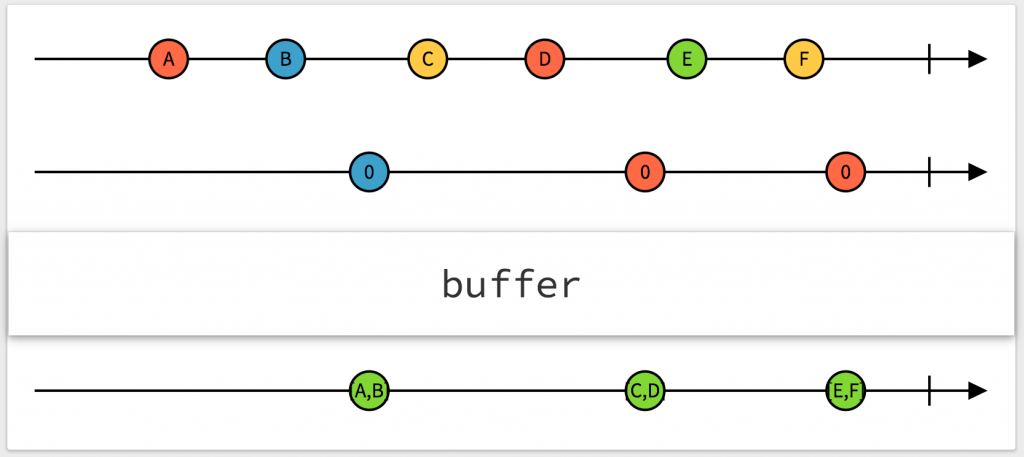
The topmost line represents the source$ Observable and the line below is the inner observable. The buffer operator emits the collected values as soon as it receives notification from the inner observable. In short, a buffer operator Buffers Observable values.
buffer<T>(closingNotifier: Observable<any>): OperatorFunction<T, T[]>
Example of RxJS buffer operator
The below code demonstrates the usage of the buffer operator. The outer observable produces data in 500ms, this is cached till 2seconds. Every 2 seconds, the buffer emits the stored values from source observable interval(500)
as an array. Note, the take(5)
operator limits the loop to 5 only. (Try on stackblitz.com)
import { interval } from "rxjs"; import { buffer, take } from "rxjs/operators"; interval(500) //source observable .pipe( buffer(interval(2000)), //inner observable take(5)//do for 5 times ).subscribe(data => console.log(data));
Output
[0, 1, 2] [3, 4, 5, 6] [7, 8, 9, 10] [11, 12, 13, 14] [15, 16, 17, 18]
The below code represents another example of buffer operator. It collects the emitting of values until the mouse click takes place. This is the kind of code you will write when you design games etc. Try it on Stackblitz.com.
import { fromEvent, interval } from "rxjs"; import { buffer } from "rxjs/operators"; const clicks$ = fromEvent(document, "click"); const bufferedObs$ = interval(1000).pipe(buffer(clicks$)); bufferedObs$.subscribe(d => console.log(d));
Output
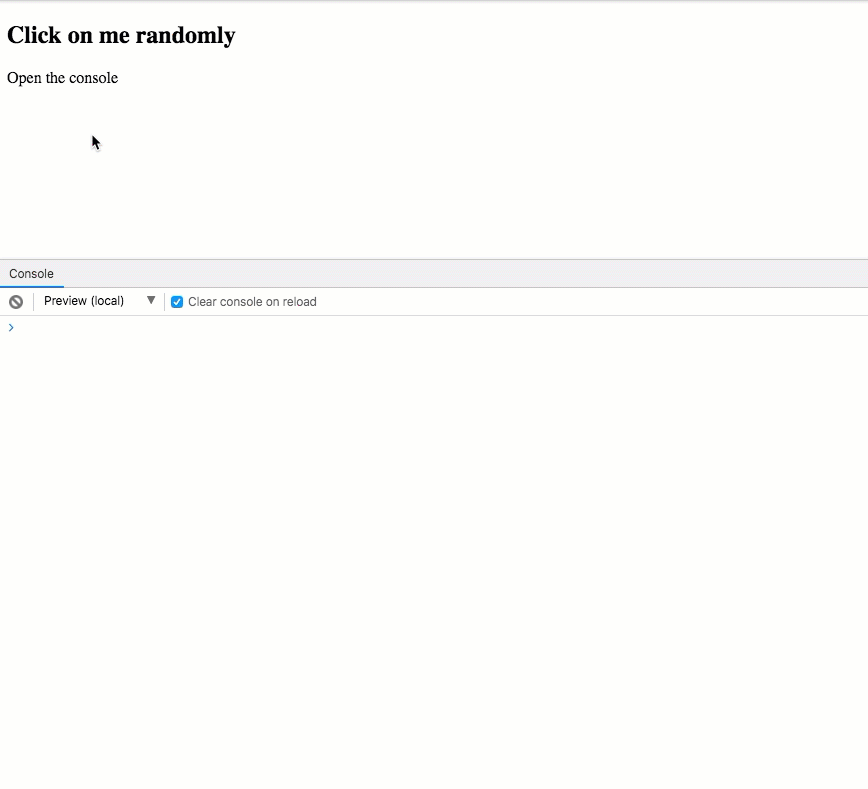
Further reading
- Check out the Game Loop example on Stackblitz.com
- Official docs on buffer operator
This is all as part of the buffer operator, please share your thoughts in the comments below.